Okay, so I’ve been messing around with this “grade” thing in Ruby, and I gotta say, it’s pretty neat. I wanted to see if I could build something simple to, you know, calculate grades, and it turned out to be a fun little project. So, here’s how it went down:
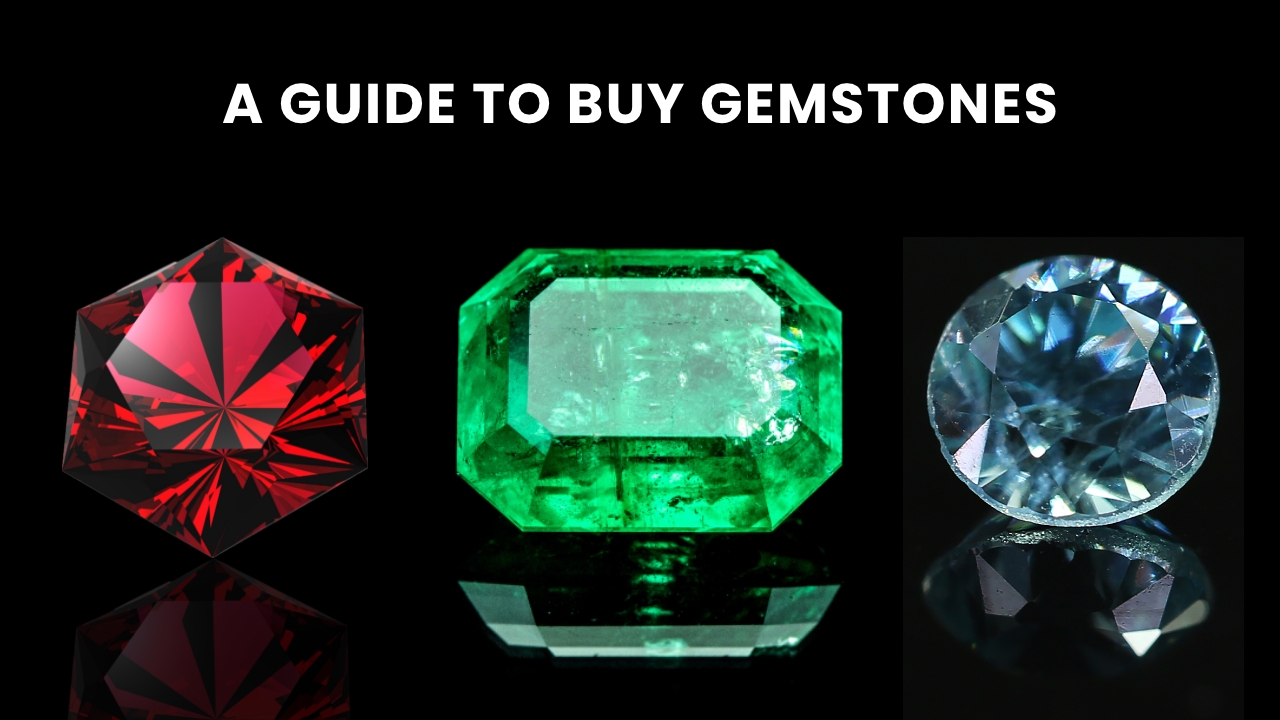
Starting Simple
First, I just wanted to get a feel for it. I fired up my text editor and started a new Ruby file. I figured, let’s start with the basics – just taking a score and figuring out if it’s a pass or a fail. I wrote some super basic code, basically an “if” statement. Something like, “if the score is greater than or equal to, say, 60, then it’s a pass, otherwise it’s a fail”.
Adding More Grades
That was cool, but kinda boring. I mean, just pass or fail? So I thought, let’s add some actual letter grades – you know, A, B, C, D, and F. This meant adding more “if” conditions, or rather, “elsif” conditions in Ruby. It got a little longer, but it was still pretty straightforward. Each “elsif” checked for a different score range, assigning the appropriate letter grade.
Making it a Function
Now, I had this chunk of code that could calculate grades, but it was just sitting there. I wanted to be able to reuse it, so I decided to wrap it up in a function. In Ruby, you use “def” to define a function. I named it something like “calculate_grade”, and it took the score as an input. Inside the function, I just pasted my “if/elsif” block. Now I could just call this function with any score and get the letter grade back.
Testing it Out
Of course, I had to make sure it was actually working right. I started calling my “calculate_grade” function with different scores – some high, some low, some right on the edge of the grade boundaries. I printed out the results to the console just to see what was happening. It’s always good to see it in action, you know?
Looping Through Scores
Then I thought, what if I had a bunch of scores? I didn’t want to call the function manually for each one. So, I used a loop! I created an array of scores, and then used a “each” loop in Ruby to go through each score in the array. Inside the loop, I called my “calculate_grade” function and printed the result. It was pretty satisfying to see all the grades pop up on the screen.
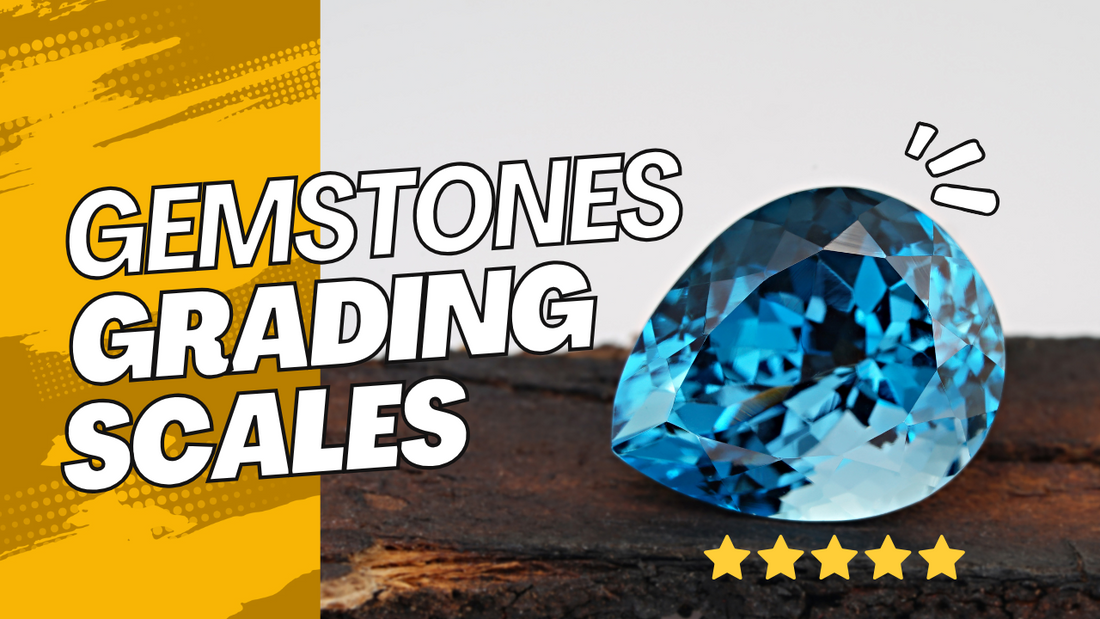
Making it Interactive
Finally made it a little more interactive. Used some build-in function that to get input from the user(that would be me). Type in a score, hit enter, and boom – there’s the grade.
It is a nice touch, feels more like a real(albeit tiny)program.
So yeah, that’s pretty much it. It’s a simple project, but it was a good way to play around with some basic Ruby concepts – “if” statements, functions, loops, and user input. And hey, now I have a little tool to calculate grades! Might come in handy someday, who knows?